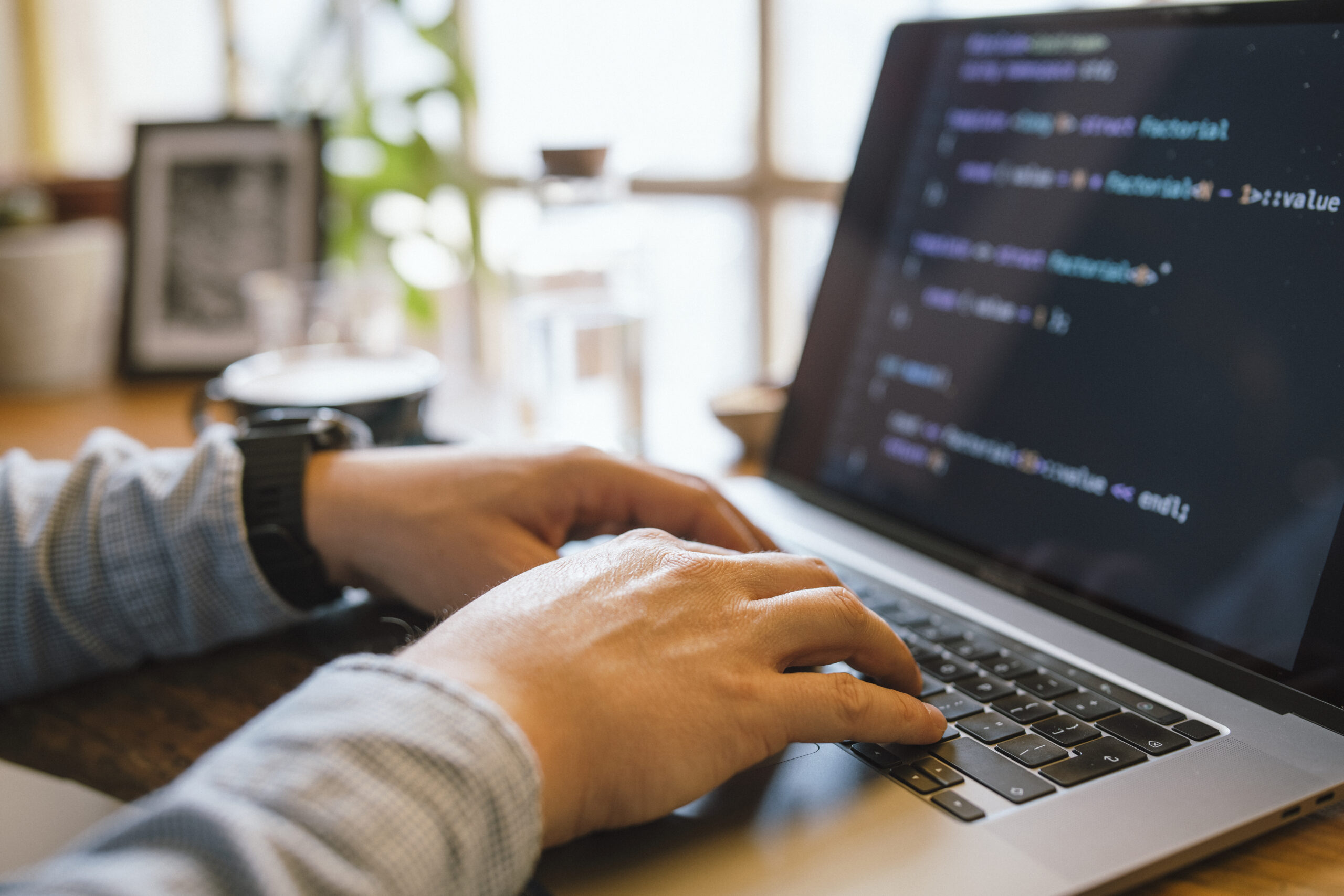
Debugging is Just about the most crucial — nonetheless often ignored — abilities within a developer’s toolkit. It is not almost repairing damaged code; it’s about knowledge how and why items go Mistaken, and Mastering to Assume methodically to unravel challenges competently. Regardless of whether you're a novice or even a seasoned developer, sharpening your debugging expertise can preserve hrs of annoyance and considerably transform your productiveness. Allow me to share many approaches that will help builders degree up their debugging recreation by me, Gustavo Woltmann.
Master Your Tools
On the list of quickest means builders can elevate their debugging capabilities is by mastering the resources they use each day. While crafting code is one Element of progress, being aware of the best way to interact with it correctly during execution is Similarly crucial. Modern enhancement environments arrive equipped with potent debugging abilities — but several developers only scratch the floor of what these resources can perform.
Get, as an example, an Integrated Growth Atmosphere (IDE) like Visible Studio Code, IntelliJ, or Eclipse. These resources allow you to established breakpoints, inspect the value of variables at runtime, move by means of code line by line, and even modify code to the fly. When utilized properly, they Enable you to observe just how your code behaves during execution, and that is invaluable for monitoring down elusive bugs.
Browser developer resources, for instance Chrome DevTools, are indispensable for front-conclusion builders. They let you inspect the DOM, observe community requests, check out authentic-time efficiency metrics, and debug JavaScript in the browser. Mastering the console, resources, and community tabs can switch disheartening UI troubles into manageable jobs.
For backend or method-stage developers, equipment like GDB (GNU Debugger), Valgrind, or LLDB offer deep Management more than jogging procedures and memory management. Mastering these equipment can have a steeper Studying curve but pays off when debugging functionality challenges, memory leaks, or segmentation faults.
Beyond your IDE or debugger, grow to be snug with version Handle programs like Git to understand code background, uncover the precise second bugs ended up released, and isolate problematic variations.
Ultimately, mastering your tools indicates heading further than default configurations and shortcuts — it’s about developing an intimate knowledge of your development atmosphere to make sure that when challenges crop up, you’re not lost in the dark. The better you know your tools, the more time you'll be able to devote fixing the actual issue instead of fumbling via the method.
Reproduce the trouble
Just about the most vital — and often overlooked — ways in helpful debugging is reproducing the issue. Before leaping in the code or generating guesses, developers need to produce a dependable ecosystem or circumstance in which the bug reliably appears. Without the need of reproducibility, repairing a bug turns into a recreation of opportunity, often bringing about wasted time and fragile code changes.
Step one in reproducing an issue is collecting just as much context as is possible. Inquire questions like: What steps led to The difficulty? Which ecosystem was it in — growth, staging, or manufacturing? Are there any logs, screenshots, or mistake messages? The more element you've got, the a lot easier it becomes to isolate the precise conditions underneath which the bug occurs.
When you finally’ve collected plenty of info, try to recreate the situation in your local natural environment. This could indicate inputting the same knowledge, simulating related person interactions, or mimicking program states. If The difficulty appears intermittently, take into account crafting automated tests that replicate the edge scenarios or state transitions concerned. These assessments not only support expose the condition but additionally protect against regressions in the future.
At times, The problem may be surroundings-precise — it would come about only on sure operating techniques, browsers, or underneath particular configurations. Utilizing applications like virtual machines, containerization (e.g., Docker), or cross-browser screening platforms might be instrumental in replicating these types of bugs.
Reproducing the problem isn’t only a phase — it’s a mindset. It demands persistence, observation, plus a methodical tactic. But as you can consistently recreate the bug, you're by now midway to repairing it. That has a reproducible state of affairs, You may use your debugging applications more effectively, take a look at opportunity fixes properly, and connect extra Evidently with all your workforce or buyers. It turns an abstract criticism right into a concrete obstacle — Which’s the place developers thrive.
Read and Realize the Error Messages
Error messages are often the most beneficial clues a developer has when a little something goes Erroneous. In lieu of looking at them as discouraging interruptions, builders must discover to take care of mistake messages as direct communications in the program. They frequently show you just what exactly took place, exactly where it happened, and in some cases even why it took place — if you understand how to interpret them.
Commence by reading the information meticulously and in whole. A lot of developers, especially when underneath time strain, look at the 1st line and instantly start building assumptions. But deeper in the mistake stack or logs might lie the legitimate root lead to. Don’t just duplicate and paste error messages into search engines like google — browse and realize them very first.
Crack the error down into sections. Is it a syntax mistake, a runtime exception, or perhaps a logic mistake? Will it place to a particular file and line range? What module or perform activated it? These concerns can tutorial your investigation and point you toward the responsible code.
It’s also valuable to understand the terminology on the programming language or framework you’re using. Error messages in languages like Python, JavaScript, or Java generally adhere to predictable designs, and Studying to acknowledge these can greatly quicken your debugging course of action.
Some errors are vague or generic, and in Those people instances, it’s very important to examine the context during which the mistake happened. Check connected log entries, enter values, and up to date adjustments from the codebase.
Don’t ignore compiler or linter warnings either. These usually precede more substantial challenges and supply hints about possible bugs.
Eventually, mistake messages are not your enemies—they’re your guides. Understanding to interpret them accurately turns chaos into clarity, serving to you pinpoint challenges faster, decrease debugging time, and become a additional economical and self-assured developer.
Use Logging Sensibly
Logging is one of the most potent resources within a developer’s debugging toolkit. When employed properly, it offers serious-time insights into how an software behaves, encouraging you have an understanding of what’s going on underneath the hood while not having to pause execution or action from the code line by line.
A good logging strategy starts off with recognizing what to log and at what amount. Prevalent logging degrees include things like DEBUG, Data, WARN, ERROR, and FATAL. Use DEBUG for comprehensive diagnostic information during enhancement, Facts for normal functions (like productive start off-ups), WARN for potential issues that don’t crack the appliance, ERROR for precise challenges, and Deadly once the method can’t continue.
Stay clear of flooding your logs with abnormal or irrelevant information. Too much logging can obscure vital messages and slow down your method. Give attention to key situations, condition changes, enter/output values, and demanding conclusion factors in your code.
Structure your log messages Plainly and regularly. Involve context, including timestamps, ask for IDs, and function names, so it’s much easier to trace troubles in dispersed programs or multi-threaded environments. Structured logging (e.g., JSON logs) might make it even much easier to parse and filter logs programmatically.
For the duration of debugging, logs let you observe how variables evolve, what conditions are fulfilled, and what branches of logic are executed—all without halting the program. They’re Primarily precious in manufacturing environments wherever stepping via code isn’t doable.
Additionally, use logging frameworks and equipment (like Log4j, Winston, or Python’s logging module) that support log rotation, filtering, and integration with monitoring dashboards.
In the long run, wise logging is about equilibrium and clarity. Having a properly-assumed-out logging method, you may reduce the time it will take to identify challenges, achieve further visibility into your applications, and improve the Total maintainability and trustworthiness of your code.
Feel Just like a Detective
Debugging is not merely a technological process—it is a method of investigation. To successfully recognize and deal with bugs, builders will have to method the method just like a detective resolving a secret. This state of mind aids break down intricate difficulties into workable pieces and follow clues logically to uncover the root bring about.
Get started by accumulating proof. Think about the symptoms of the issue: error messages, incorrect output, or overall performance problems. Much like a detective surveys against the law scene, accumulate just as much suitable facts as you may without the need of leaping to conclusions. Use logs, take a look at scenarios, and consumer experiences to piece alongside one another a transparent photo of what’s taking place.
Up coming, type hypotheses. Inquire your self: What could be causing this actions? Have any modifications recently been built into the codebase? Has this challenge transpired just before below similar instances? The target should be to more info slim down prospects and identify opportunity culprits.
Then, take a look at your theories systematically. Make an effort to recreate the issue inside of a managed atmosphere. If you suspect a specific purpose or element, isolate it and validate if The problem persists. Like a detective conducting interviews, check with your code queries and let the final results lead you nearer to the reality.
Spend shut focus to small facts. Bugs frequently disguise inside the the very least anticipated places—similar to a missing semicolon, an off-by-a person error, or simply a race issue. Be complete and individual, resisting the urge to patch The difficulty with no fully comprehension it. Short term fixes may perhaps conceal the actual problem, just for it to resurface afterwards.
Finally, retain notes on Everything you tried out and learned. Equally as detectives log their investigations, documenting your debugging method can help you save time for potential difficulties and assist Other folks understand your reasoning.
By pondering just like a detective, builders can sharpen their analytical abilities, strategy challenges methodically, and become simpler at uncovering concealed challenges in complicated programs.
Produce Checks
Creating exams is one of the simplest methods to boost your debugging capabilities and In general development efficiency. Tests not just aid capture bugs early and also function a security Web that gives you confidence when creating adjustments to the codebase. A properly-examined software is simpler to debug as it helps you to pinpoint exactly where and when a problem occurs.
Get started with device checks, which deal with unique capabilities or modules. These smaller, isolated assessments can speedily expose irrespective of whether a selected bit of logic is Doing work as anticipated. Whenever a check fails, you immediately know where to glimpse, noticeably cutting down the time used debugging. Device exams are Particularly useful for catching regression bugs—challenges that reappear just after Earlier getting set.
Next, combine integration assessments and conclude-to-finish tests into your workflow. These enable be certain that different parts of your software perform together effortlessly. They’re specifically helpful for catching bugs that happen in elaborate programs with several factors or companies interacting. If some thing breaks, your checks can let you know which part of the pipeline unsuccessful and under what ailments.
Creating tests also forces you to definitely think critically regarding your code. To test a attribute correctly, you require to comprehend its inputs, envisioned outputs, and edge instances. This standard of knowing The natural way sales opportunities to better code construction and much less bugs.
When debugging an issue, composing a failing exam that reproduces the bug could be a robust starting point. After the take a look at fails consistently, it is possible to deal with repairing the bug and check out your check move when The difficulty is resolved. This strategy makes sure that the same bug doesn’t return Later on.
In a nutshell, crafting tests turns debugging from a aggravating guessing game into a structured and predictable method—serving to you capture more bugs, more quickly and a lot more reliably.
Choose Breaks
When debugging a tough problem, it’s straightforward to become immersed in the situation—gazing your display for hours, making an attempt Resolution immediately after Alternative. But one of the most underrated debugging tools is simply stepping away. Taking breaks assists you reset your thoughts, minimize disappointment, and infrequently see The difficulty from the new point of view.
When you are far too near to the code for way too prolonged, cognitive tiredness sets in. You could possibly start off overlooking clear problems or misreading code that you just wrote just hrs before. With this condition, your brain turns into much less effective at problem-resolving. A brief stroll, a coffee crack, or simply switching to a unique process for ten–quarter-hour can refresh your target. Numerous builders report acquiring the basis of an issue after they've taken the perfect time to disconnect, allowing their subconscious perform within the history.
Breaks also enable avert burnout, Specifically in the course of lengthier debugging classes. Sitting in front of a display screen, mentally caught, is not only unproductive and also draining. Stepping away allows you to return with renewed Electricity plus a clearer state of mind. You may perhaps out of the blue observe a missing semicolon, a logic flaw, or maybe a misplaced variable that eluded you just before.
If you’re caught, a great guideline would be to established a timer—debug actively for 45–sixty minutes, then have a 5–ten minute split. Use that time to maneuver about, extend, or do some thing unrelated to code. It could really feel counterintuitive, In particular below restricted deadlines, but it in fact leads to more rapidly and more practical debugging Over time.
To put it briefly, taking breaks is not really a sign of weak point—it’s a sensible technique. It offers your Mind Area to breathe, enhances your standpoint, and assists you stay away from the tunnel eyesight That always blocks your progress. Debugging can be a psychological puzzle, and relaxation is part of resolving it.
Learn From Each and every Bug
Just about every bug you encounter is more than just A short lived setback—It is really an opportunity to expand for a developer. Whether it’s a syntax error, a logic flaw, or even a deep architectural situation, every one can instruct you one thing precious for those who make an effort to reflect and examine what went Mistaken.
Start out by inquiring you a few important queries after the bug is settled: What induced it? Why did it go unnoticed? Could it are caught before with superior methods like unit testing, code reviews, or logging? The answers often reveal blind places in the workflow or understanding and help you build stronger coding patterns going ahead.
Documenting bugs can even be a fantastic routine. Hold a developer journal or retain a log in which you Notice down bugs you’ve encountered, how you solved them, and Everything you learned. Over time, you’ll begin to see designs—recurring concerns or typical mistakes—that you can proactively stay clear of.
In staff environments, sharing Whatever you've realized from a bug with all your friends might be Specifically potent. Whether it’s via a Slack concept, a brief produce-up, or a quick awareness-sharing session, serving to Other individuals avoid the similar concern boosts team performance and cultivates a more powerful Discovering lifestyle.
More importantly, viewing bugs as classes shifts your frame of mind from aggravation to curiosity. In lieu of dreading bugs, you’ll start off appreciating them as essential portions of your improvement journey. In fact, a number of the best developers are usually not the ones who produce excellent code, but individuals that constantly study from their errors.
In the long run, Every bug you deal with adds a different layer for your ability established. So subsequent time you squash a bug, have a instant to reflect—you’ll arrive absent a smarter, more capable developer as a consequence of it.
Summary
Enhancing your debugging techniques takes time, apply, and endurance — but the payoff is huge. It can make you a far more effective, self-confident, and able developer. Another time you're knee-deep within a mysterious bug, recall: debugging isn’t a chore — it’s a possibility to become much better at Whatever you do.